August 8, 2012
by Cennest
Drag and Drop is a very useful and common feature. This allows you to drag an object to a location and drop the object there, with the help of a mouse click.
To achieve drag and drop functionality with traditional HTML4, developers would either have to use complex Javascript programming or other Javascript frameworks like flash etc.
Now HTML 5 has come up with a Drag and Drop(dnd) API that brings native a dnd support to the browser making it much easier to code up.
Html 5 Drag and Drop is supported by all major browsers – Internet Explorer 9, Firefox, Opera 12, Chrome support drag and drop.
Drag and Drop Events:
There are number of events which are fired during various stages of the drag and drop operation. These events are listed below:
Events
|
Description
|
dragstart
|
Fires when the user starts dragging the object.
|
dragenter
|
Fired when the mouse is first moved over the target element while a drag is occurring. A listener for this event should indicate whether a drop is allowed over this location. If there are no listeners, or the listeners perform no operations, then a drop is not allowed by default.
|
dragover
|
This event is fired as the mouse is moved over an element when a drag is occurring. Much of the time, the operation that occurs during a listener will be the same as the dragenter event.
|
dragleave
|
This event is fired when the mouse leaves an element while a drag is occurring. Listeners should remove any highlighting or insertion markers used for drop feedback.
|
drag
|
Fires every time the mouse is moved while the object is being dragged.
|
drop
|
The drop event is fired on the element where the drop was occurred at the end of the drag operation. A listener would be responsible for retrieving the data being dragged and inserting it at the drop location.
|
dragend
|
Fires when the user releases the mouse button while dragging an object.
|
In this article, we’ll create a very simple example of an HTML5 drag and drop.
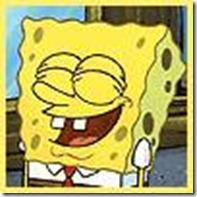
We’ll be dragging and dropping this image.
Html Code:DragandDrop.html
<!DOCTYPE html>
<html lang="en">
<head>
<title>Html5 Drag and Drop Demo</title>
<link rel="stylesheet" type="text/css" href="Content/DragandDrop.css">
<script type="text/javascript" src="Scripts/DragandDrop.js"></script>
</head>
<body>
<div id="content">
<section id="topBox">
<!–In Html5 we don’t need to add an ending tag for .css and img tag–>
<img id="image1" src="Images/image1.gif">
</section>
<section id="bottomBox">
Drag the image here!
</section>
</div>
</body>
</html>
Css Code:DragandDrop.css
#content {
width:400px;
margin:0 auto;
padding:30px;
}
#topBox
{
width:400px;
height:100px;
margin:5px;
border:2px solid #000000;
}
#bottomBox
{
width:400px;
height:300px;
margin:5px;
border:2px solid #0094ff;
}
1. Make an element draggable, by setting the draggable attribute to true. Here, I’ll be dragging an image so I am setting the draggable property of the image to true.
<img id="image1" src="Images/image1.gif" draggable="true" />
2. Write function() when web page has finished loading by calling
window.addEventListener("load", OnLoad, false);
in DragAndDrop.js
JS code:DragandDrop.js
window.addEventListener("load", OnLoad, false);
When window is loaded we’ll call OnLoad() method.
function OnLoad() {
//get the element with id’image1′ and store it in variable pic1.
pic1 = document.getElementById(‘image1’);
//When we start dragging the element,dragstart event is fired and StartDrag function //is called.
pic1.addEventListener("dragstart", StartDrag, false);
//bottomBox is the id of element where the image has to be dragged to.
bottomBox = document.getElementById(‘bottomBox’);
//dragenter event is fired when the image is dragged to the bottomBox and DragEnter //function is called. Inside the function we prevent the default action for the event //as it may differ in each browser.’e’ refers to event
bottomBox.addEventListener("dragenter", function (e) { e.preventDefault(); }, false);
//dragover event is fired when the image is dragged over the bottomBox and the //respective function is called.Inside the function we prevent the default action for //the event as it may differ in each browser.’e’ refers to event.
bottomBox.addEventListener("dragover", function (e) { e.preventDefault(); }, false);
//drop event is called when the image is dropped in the bottomBox and Dropped function //is called.
bottomBox.addEventListener("drop", Dropped , false);
}
//This function is called when dragstart event is fired. ‘e’ refers to event.An event //is some action that the user can do on a webpage.e.g dragging is an event.
//When dragstart event occurs it stores the information of image source code.
function StartDrag(e) {
//image source code is stored in a variable code.
var code = ‘ <img id="image1" src="Images/image1.gif" draggable="true">’;
//dataTransfer.setData() is used for storing information on the event. This method
//sets the data type and the value of the dragged data.
e.dataTransfer.setData(‘Text’, code);
}
Here, the data type is "Text" and the value is the source code of the draggable element .
function Dropped(e) {
//Call preventDefault() to prevent the browser default handling of the data (default is //open as link on drop)
e.preventDefault();
//Get the dragged data with the dataTransfer.getData("Text") method. This method will //return any data that was set to the same type in the setData() method.
//Here, the content of bottomBox changes to an image.
bottomBox.innerHTML = e.dataTransfer.getData(‘Text’);
}
Here, the dragged data is the source code of the dragged element.
This is how it looks once you have dragged the image to the botom box.
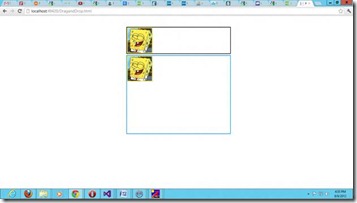
Further Improvement.
In this we will make the upper image disappear once the dragging and dropping of image is complete.
JS Code: DragandDrop.js
function OnLoad() {
//get the element with id’image1′ and store it in pic1.
pic1 = document.getElementById(‘image1’);
//When we start dragging the element StartDrag function is called
pic1.addEventListener("dragstart", StartDrag, false);
//EndDrag function is called when its done with dragging the image.
pic1.addEventListener("dragend", EndDrag, false);
//bottomBox is the id of element where the image has to be dragged to.
bottomBox = document.getElementById(‘bottomBox’);
//dragenter event is fired when the image is dragged to the bottomBox and DragEnter //function is called.
bottomBox.addEventListener("dragenter", DragEnter, false);
//dragleave event is fired when the image is dragged to the bottomBox but has not yet //dropped the image and then leaves the bottomBox.
//DragLeave function is called.
bottomBox.addEventListener("dragleave", DragLeave, false);
//dragover event is fired when the image is dragged over the bottomBox and the //respective function is called.
//Inside the function we prevent the default action for the event as it may differ in each browser.’e’ refers to event
bottomBox.addEventListener("dragover", function (e) { e.preventDefault(); }, false);
//drop event is called when the image is dropped in the bottomBox and Dropped function is called.
bottomBox.addEventListener("drop", Dropped , false);
}
//This function is called when dragstart event is fired.’e’ is event.
//An event is something that the user can do on a webpage.e.g dragging is an event.
//When dragstart event occurs it stores the information of image source code.
function StartDrag(e) {
//image source code is stored in a variable code.
var code = ‘ <img id="image1" src="Images/image1.gif" draggable="true">’;
//dataTransfer.setData() is used for storing information on the event. This method
//sets the data type and the value of the dragged data.
e.dataTransfer.setData(‘Text’, code);
}
function EndDrag(e) {
//Event is dragging and target of event is image here.
pic = e.target;
//once the dragging is complete the visibility of the image in the topBox is hidden.
pic.style.visibility = ‘hidden’;
}
function DragEnter(e) {
e.preventDefault();
//When the image enters the bottomBox its background color and border color changes.
bottomBox.style.backgroundColor = ‘SkyBlue’;
bottomBox.style.border = ‘2px solid green’;
}
function DragLeave(e) {
e.preventDefault();
//When the image leaves the bottomBox and the image has not been dropped yet its background color and border color changes.
bottomBox.style.backgroundColor = ‘White’;
bottomBox.style.border = ‘2px solid #0094ff’;
}
function Dropped(e) {
//Call preventDefault() to prevent the browser default handling of the data (default is open as link on drop)
e.preventDefault();
//Get the dragged data with the dataTransfer.getData("Text") method.
//This method will return any data that was set to the same type in the setData() method.
bottomBox.innerHTML = e.dataTransfer.getData(‘Text’);
}
This is how it looks once you have dragged the image to the bottom box.
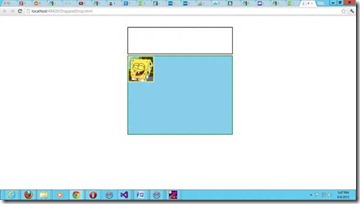
You can download the zipped up code for this example from here.
Hope this helps !!
Team Cennest !!
References
http://www.tutorialspoint.com/html5/html5_drag_drop.htm
http://www.w3schools.com/html5/html5_draganddrop.asp
http://www.youtube.com/watch?v=Kfi3uRJS6hA
http://www.youtube.com/watch?feature=fvwp&NR=1&v=-2qhd7uJkn8
http://www.youtube.com/watch?feature=fvwp&NR=1&v=SWR5ojeSmvM
http://www.youtube.com/watch?feature=fvwp&NR=1&v=e6t-8EoT2HM